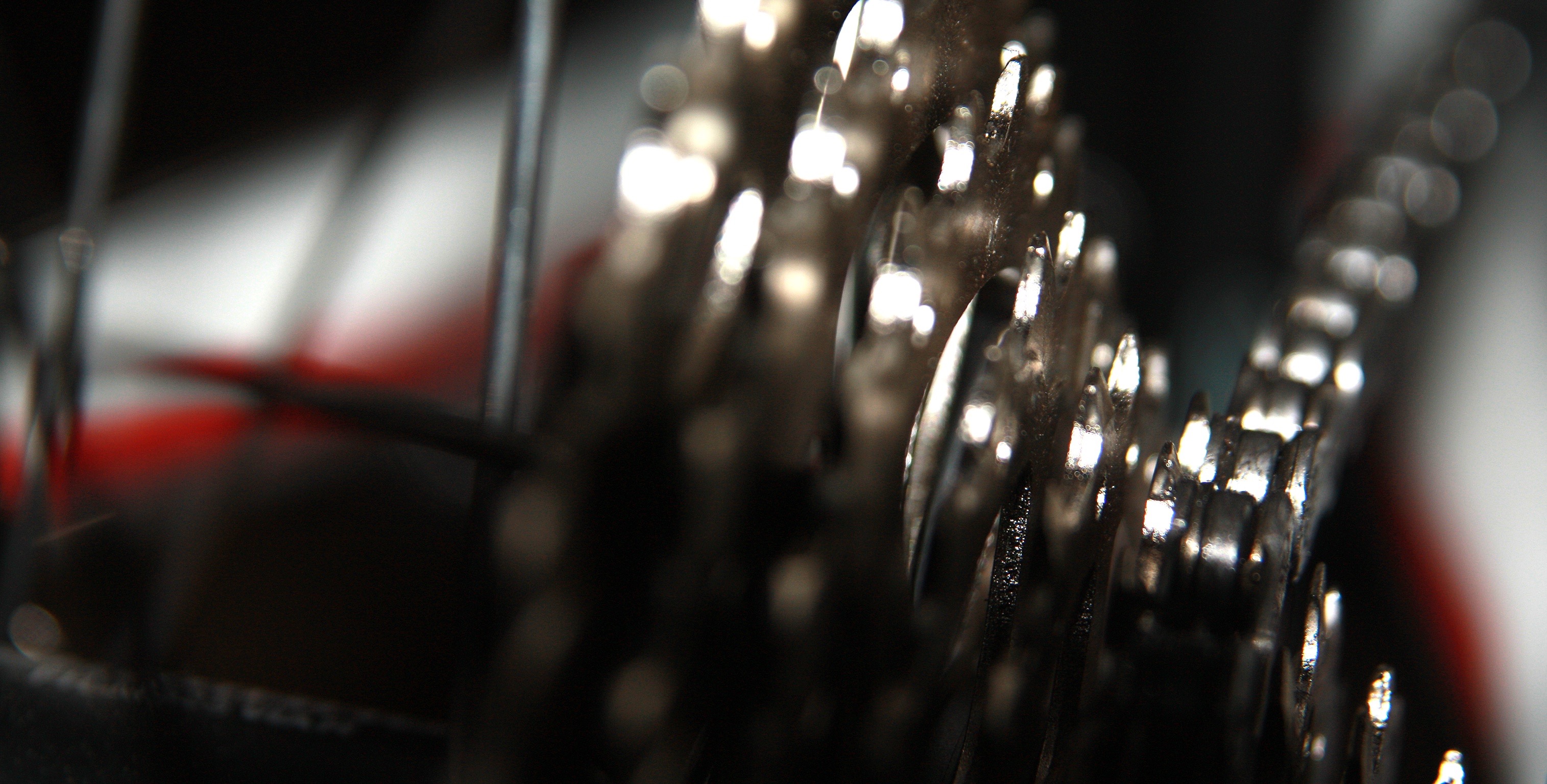
first python steps with argparser
my first python script - just a proof of concept to parse parameter
#!/usr/bin/python
import argparse
import os
argp = argparse.ArgumentParser(description="ping desired hostname / ip as often you want",epilog="just a proof of concept...")
argp.add_argument('-H','--host',help='target hostname / ip',required=True)
argp.add_argument('-C','--count',help='how many pings',default=2)
args = argp.parse_args()
os.system("ping -c " + str(args.count) + " " + args.host)
so you get a nice help message and usage output
[root@host] ~ # ./monty.py
usage: monty.py [-h] -H HOSTNAME [-C COUNT]
monty.py: error: argument -H/--hostname is required
[root@host] ~ # ./monty.py -h
usage: monty.py [-h] -H HOSTNAME [-C COUNT]
ping desired hostname / ip as often you want
optional arguments:
-h, --help show this help message and exit
-H HOSTNAME, --hostname HOSTNAME
target hostname / ip
-C COUNT, --count COUNT
how many pings
just a proof of concept...
[root@host] ~ #
deactivate touchpad on an Lenovo laptop
to deactivate a touchpad on a Lenovo laptop just run the following script
#!/bin/bash
# get ID from Touchpad
ID=$(xinput list | grep TouchPad | awk '{ print $6 }' | awk -F= '{ print $2 }')
# deactivate the Touchpad
xinput set-prop ${ID} "Device Enabled" 0
if [ $? != 0 ]; then
echo "[FAIL] TouchPad is still active!"
exit 1
else
echo "[ok] TouchPad is deactivated!"
fi
exit 0
RasPi Hardware Random Number Generator
the raspberry pi comes with a built in random number generator chip. to activate the chip and use it as your default random-source, just follow the few steps:
- add the line ''bcm2708_rng'' to /etc/modules
- activate the device and install a toolset which alows you to use random numers systemwide.
- change the config ''/etc/default/rng-tools'' AND restart the service.
this little script will help you
#!/bin/bash
# inst_hwrng.sh
echo "bcm2708_rng" >> /etc/modules
/dev/hwrng
apt-get update
apt-get install -y rng-tools
sed -i 's/\#HRNGDEVICE\=\/dev\/null/HRNGDEVICE=\/dev\/hwrng/g' /etc/default/rng-tools
/etc/init.d/rng-tools restart
Timelaps with RasPi-Cam
with a camera for your raspberry pi, you simple make pictures with raspistill -o name.jpg So why not put this in a loop and make a timelaps video?
#!/bin/bash
# bash-script for raspberry pi with camera to make time laps photos
# need to install mathematic-library bc! "apt-get update && apt-get install bc"
# to put the pictures together to a movie I use iMovie...
log_date="$( date +%Y%m%d-%H%M )"
log_dir="/srv/logs/"
log_file="${log_dir}timelaps-${log_date}.log"
pic_dir="/mnt/user/timelaps/"
log() {
echo "$(date +%Y.%m.%d-%H:%M:%S)" "$1" >> ${log_file};
echo $1
}
read -p "in one word, why?: " why
dest="${pic_dir}${why}/"
if [ ! -d $pic_dir ]; then
echo "[FAIL] mountpoint does not exist! EXIT..."
exit 1
else
if [ ! -d $dest ]; then
echo "[ok]"
else
echo "[FAIL] reason already exists! EXIT..."
exit 1
fi
fi
read -p "how long would you like to capture? (min): " cap_time
echo "checking space on ${pic_dir}..."
pic_max=$(expr $cap_time \* 6)
pic_space=$(bc <<< "$pic_max*2.9" | awk -F'.' '{ print $1 }')
space_need=$(bc <<< "$pic_max*2.9*1024" | awk -F'.' '{ print $1 }')
space_left=$(df ${pic_dir} | grep ^/ | awk '{ print $4 }')
if [[ ${space_left} < ${space_need} ]]; then
echo "[ok]"
echo "creating directory ${why}"
/bin/mkdir ${dest}
if [ "$?" -ne "0" ]; then
echo "[FAIL] could not create ${dest}! EXIT..."
exit 1
else
echo "[ok] logfile will be written to ${log_file}"
fi
else
echo "[FAIL] not enough space on device! EXIT..."
exit 1
fi
log "START captureing Timelaps ${why}"
log "${pic_max} files will be created as: ${dest}[xx].jpg"
log "${pic_space} MB space is needed..."
for i in $(eval echo "{1..$pic_max}"); do
log "..4..3..2..1..smile...";
sleep 4
log "takeing picture number: $i"
raspistill -o ${dest}${i}.jpg
log "<----------------------------->"
done
log "FINISHED!"
exit 0
How to use "mod_auth_dbd" and "mod_auth_form" with apache 2.4 on debian jessie
first you need to install some packages:
apt-get install apache2 apache2-utils mysql-server
apt-get install libaprutil1-dbd-mysql
create mysql-user: authtest with DB authtest and add table:
CREATE TABLE `password` (
`id` int(11) unsigned NOT NULL auto_increment,
`username` varchar(255) default NULL,
`password` varchar(255) default NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
create user and pass for authentication and add to table:
htpasswd -bns User pass
User:{SHA}nU4eI71bcnBGqeO0t9tXvY1u5oQ=
INSERT INTO `password` (`username`, `password`) VALUES('User', '{SHA}nU4eI71bcnBGqeO0t9tXvY1u5oQ=');
create /etc/apache2/conf-available/dbd_mysql.conf:
## mod_dbd configuration
DBDriver mysql
DBDParams "dbname=authtest user=authtest pass=foobar"
DBDMin 4
DBDKeep 8
DBDMax 20
DBDExptime 300
modify /etc/apache2/sites-enabled/000-default.conf:
## protected directory
AuthName "You Must Login"
AuthType Basic
AuthBasicProvider dbd
AuthDBDUserPWQuery "SELECT password AS password FROM password WHERE username = %s"
Require valid-user
load needed modules and restart apache:
a2enmod dbd
a2enmod authn_dbd
authn_socache
a2enconf dbd_mysql
service apache2 restart
extend with "mod_auth_form":
to use a self-written form on your webpage instead of the buit-in Browser Pop-Up to insert credentials, you need some tweaking: edit config "/etc/apache2/sites-enabled/000-default.conf":
AuthName "DBD-FORM"
AuthType form
AuthFormProvider dbd
Session On
SessionCookieName session path=/
ErrorDocument 401 /login.html
AuthDBDUserPWQuery "SELECT password AS password FROM password WHERE username = %s"
require valid-user
build a page with forms "login.html":
enable needed modules and restart apache:
a2enmod authn_core
a2enmod auth_form
a2enmod auth_request
a2enmod auth_requests
a2enmod request
a2enmod session
a2enmod session_cookie
service apache2 restart
Windows batch
Delete a directory inside the Mozilla profile for all users.
@echo off
cls
setlocal enabledelayedexpansion
for /d %%u in ("C:\Users\*") do (
set FFProfDir=%%u\AppData\Roaming\Mozilla\Firefox\Profiles
for /D %%a in ("!FFProfDir!\*") do (
if exist "%%a\extensions\$somefolder" rmdir /s /q "%%a\extensions\$somefolder"
)
)